There’s a multitude of resources online that’ll teach you to build websites with React starting from first principles. But if you want to pick up React when you already know Javascript you might find yourself wading through a lot of basics before you get to what you want to learn.
This article (and the others that follow) are basically brain dumps of things I wish I’d known before starting on my first React/Typescript project. This first one is a very high level overview of some key concepts.
Prerequisites
So what should you already know? I reckon these are probably the minimum prerequisites:
- Javascript
- HTML5
- Setting up your dev environment and installing NPM packages
- Ideally, some experience using a typed language such as C, C# or Java
I’m going to cover React in conjunction with Typescript. You don’t have to use them together but personally I think it makes for more robust code thanks to its compile time type checking. I’m not intending to regurgitate stuff you can find elsewhere so I’ll link out to documentation sources which you might want to consult.
Getting Started
The first thing you want to do is set up a basic project structure. To do this we use a package called, sensibly enough, Create React App. As per the Quickstart, run the following at your command line :
npx create-react-app my-app --template typescript
cd my-app
npm start
Two things just happened. One, we created a new React project and, two, we started a development server to run the project. That development server will keep monitoring the project directory and rebuild it any time one of your source files change.
Wait! Building?
Yep. Unlike some other Javascript frameworks you might have used previously, React projects need to be built before they can be run. Part of this involves transpiling Typescript into Javascript that the browser can run and part of it is down to Webpack which does things like minifying the output files and copying images and styles into the right directory.
When running the development server, some extra Javascript is inserted into the page which causes the browser to check back every ten seconds and hot reload the page if any of the code has changed. This is quite cool and makes for quicker iterations of coding. It also has the unfortunate side effect of creating an extra thing that can go wrong. If you’re editing your code and not seeing the changes you expect in the browser, check in on your development server process. It’s probably decided to just stop running and you’ll need to restart it. I wasted quite a lot of time thinking I was doing something wrong before I figured that one out.
Components
React works with a concept of components. Components are self-contained bits of functionality that combine presentation and function. You as developer decide how big a chunk of functionality each component represents but, in general, if you find you’re losing track of what a component is for or you’re repeating yourself, you probably need to break things down into smaller components.
A key principle of React is that we favour composition over inheritance. If you have several components that share some common functionality, you might be tempted to have them inherit from a common base. Instead, create a component that handles the common stuff and takes a more specialised component as a child.
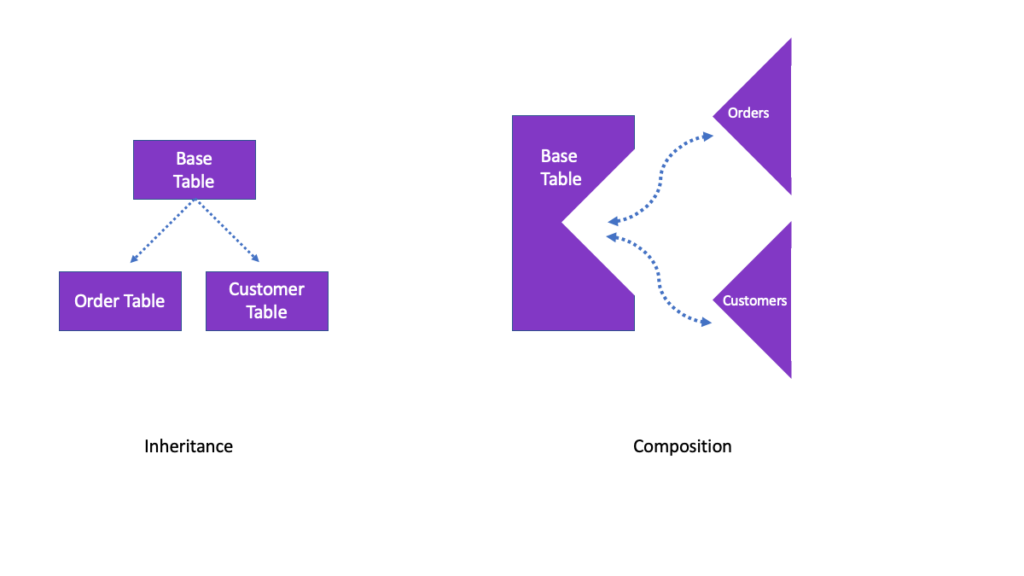
You usually pass data into components as props (there are other ways which we will discuss later). Props are just parameters but it is important to understand that they only go one way. You pass props into components. Components don’t change the props. This is not Angular JS with its two-way data binding.
So how do we get data out of the component again? We can use the usual Javascript method of calling event handlers. You can pass function references into components as props. For example, a button component can have an onClick prop which is a function that is called when the button is clicked.
JSX
You can’t do much with React without encountering JSX. It’s a way of expressing the browser DOM in Javascript via an XML-like format. Basically, it looks a lot like HTML and for most purposes you can probably treat it as HTML. One difference is that the nodes can be either HTML entities or they can be React components. And when they’re React components the attribute values are those props we talked about above.
When using HTML entities in JSX there are a couple of differences you should be aware of:
- The HTML
class
attribute is renamed asclassName
- Inline styles are expressed as Javascript objects rather than as a string
Functions, classes and functional components
A React component can be created in one of three ways : as a function, as a class or as a functional component. Pretend you haven’t heard about functions and classes. The modern way of using React is to use functional components and something called Hooks.
A functional component is just a Javascript function that, when called, outputs some JSX. When the React framework calls your function that’s known as a render. A component renders whenever its props change or when its parent component re-renders. Initially these renders take place in a Virtual DOM which is an in-memory representation of the browser DOM. Once React has finished rendering all the components, the actual browser DOM is synchronised with the virtual DOM. This makes the rendering process much faster and more efficient.
Calling a function to spit out some JSX is great but what happens when we want our component to do something more involved, like maintaining its own state? That’s where hooks come in. According to the official tutorial :
Hooks are functions that let you “hook into” React state and lifecycle features from function components
https://reactjs.org/docs/hooks-overview.html#but-what-is-a-hook
Personally, I don’t think “hooks” is a great name but it’s what we’re stuck with so let’s just run with it. Hooks are just functions that you can import into your components. By convention their names all start with the word use but apart from that there isn’t really anything special about them.
What you do need to be aware of though are the so-called “rules of hooks“. Namely:
- Only Call Hooks at the Top Level
- Only Call Hooks from React Functions
Now’s probably a good time to skim read the official overview of hooks if you haven’t already.
Things to drop-in to your project
React has a massive ecosystem of open source components, frameworks and libraries. You can quickly end up facing the paradox of choice. These are the libraries I wish I’d known about at the beginning of my React journey:
- React Router – handles single page app navigation
- React Hook Form – easy and powerful form validation
- React Query – easily fetch data from your backend servers. Transparently handles caching, refetching, long polling etc
- React Table – hooks for handling HTML tables. Bring your own UI and use the hooks to give you things like sortable, editable, filterable columns
Redux
One thing you might encounter quite a lot if you read other articles about React is Redux. For some people, React and Redux are one and the same. This is not the case. Redux is a state management library which can be really useful in large projects but for beginning React users it just adds complexity. Don’t feel pressured into using it. Even the creator of Redux says
don’t use Redux until you have problems with vanilla React.
https://redux.js.org/faq/general#when-should-i-use-redux
Next time
Coming up in subsequent posts : a quick overview of Typescript and starting to build a site with those drop-ins I mentioned above.